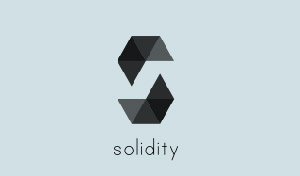
I’ve been meaning to jump back into smart contracts for a while now and thought what better time than now with so many experts around me.
Here is one of the most simplest contracts you can write in Remix, which is an amazing online Solidity IDE written by some Russian Einstein like kid.
pragma solidity ^0.4.17; contract light { event TurnLightOn(string thing); function () public { TurnLightOn("We should turn the light on"); } }
It should be self explanatory but just in case, all smart contracts need to start with the pragma first line otherwise you’ll get a compilation error. The contract is given a name called light, it generates an event and has a public fall back function. A fall back function is how The DAO hack happened. It is a default function that gets called when no function is specified. Officially “Fallback functions are triggered when the function signature does not match any of the available functions in a Solidity contract.”
This was for the hackathon so don’t use this code for real.
In remix click the details button and you’ll the commands to deploy the code.
Now comes the tricky part! You can’t just copy and paste this code because the solidity language is in flux at the moment. Lots of changes are being made and syntax has changed and lots of stuff being deprecated. Frustrating I know!
First of all the first line needs “new” and an upper case C. See below.
var browser_untitled_sol_lightContract = web3.eth.contract([{"payable":false,"stateMutability":"nonpayable","type":"fallback"},{"anonymous":false,"inputs":[{"indexed":false,"name":"thing","type":"string"}],"name":"TurnLightOn","type":"event"}]);
var browser_untitled_sol_lightContract = new web3.eth.Contract([{"payable":false,"stateMutability":"nonpayable","type":"fallback"},{"anonymous":false,"inputs":[{"indexed":false,"name":"thing","type":"string"}],"name":"TurnLightOn","type":"event"}]);
Then use this as a reference: https://web3js.readthedocs.io/en/1.0/web3-eth-contract.html#new-contract
The actual code becomes:
var Web3 = require('web3'); var web3 = new Web3('http://localhost:8545'); var testContract = new web3.eth.Contract([{"payable":false,"stateMutability":"nonpayable","type":"fallback"},{"anonymous":false,"inputs":[{"indexed":false,"name":"thing","type":"string"}],"name":"TurnLightOn","type":"event"}]); options = { data: '0x60606040523415600e57600080fd5b60a480601b6000396000f30060606040523415600e57600080fd5b7f3ee719f7fb50cecc29de7ddbf0821bdb9fa7ba9b05ceebb70bcb15cd880a912660405180806020018281038252601b8152602001807f57652073686f756c64207475726e20746865206c69676874206f6e000000000081525060200191505060405180910390a10000a165627a7a72305820b7d0e3158641982af17cdaccca45a5e5b84081e1ce26399a7be443eed176cb670029' } testContract.deploy(options) .send({ from: '0x35844071c18ba82181b5ef76269ba46b5596fb58', gas: '3000000' }) .on('transactionHash', (tx) => { console.log(tx); }) .on('receipt', (receipt) => { console.log(receipt.contractAddress); }) .on('confirmation', (confirmNumber) => { console.log(confirmNumber); }) console.log("This is proccessed");
Place this in a text file called testSmartContract.js and then execute it with:
> node testSmartContract.js
This is assuming you have testrpc up and running already. If not, check this.
What you should see is the test contract deployed, my file was called depositFund.js and you can see the contract deployed in the second terminal on the right hand side.
It takes a bit of re-learning but we got there in the end.