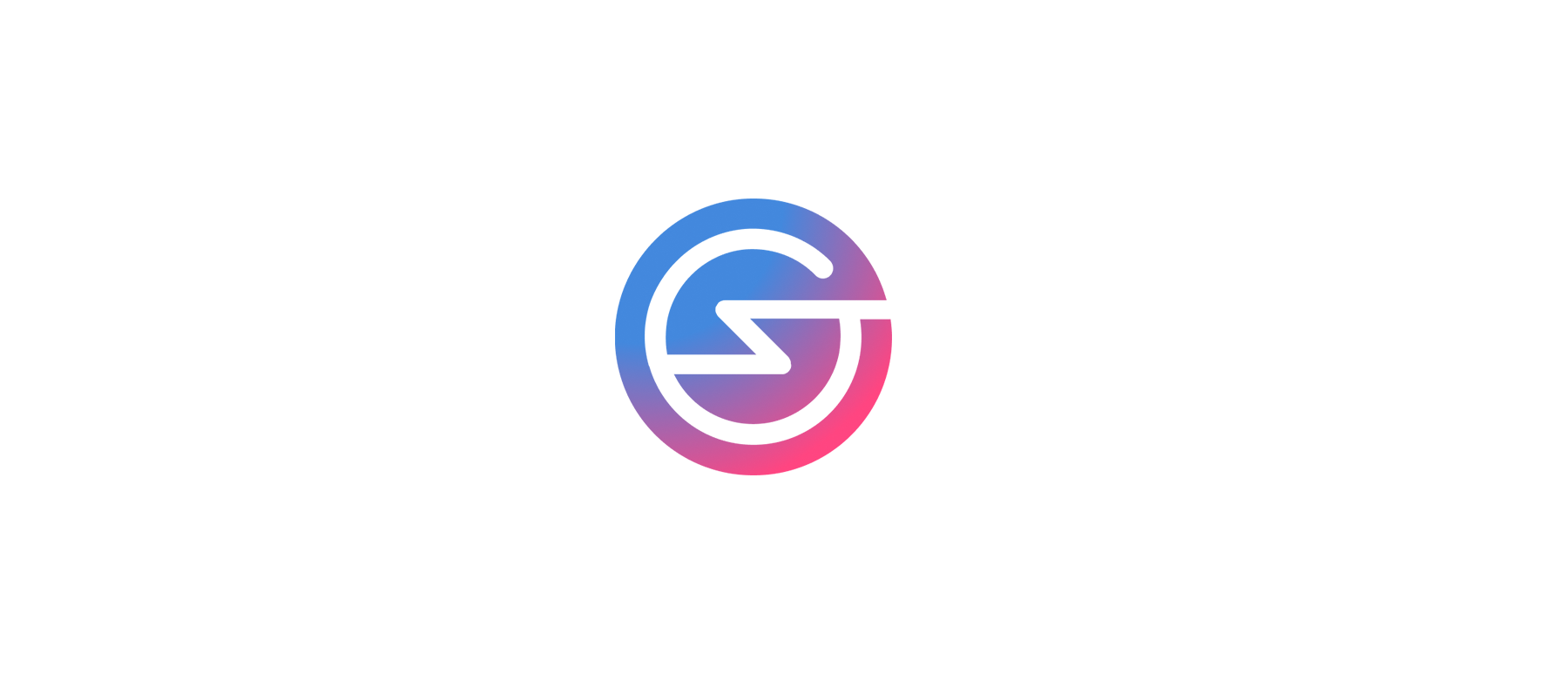
The SubQuery starter project is great way to get up and running very quickly. One drawback however is that it labels its field as “field1, field 2” etc and that it attempts to demonstrate the 3 types of mapping functions, namely block handlers, event handlers and call handers all in one go. This isn’t a bad thing but it can be slightly overwhelming for a beginner.
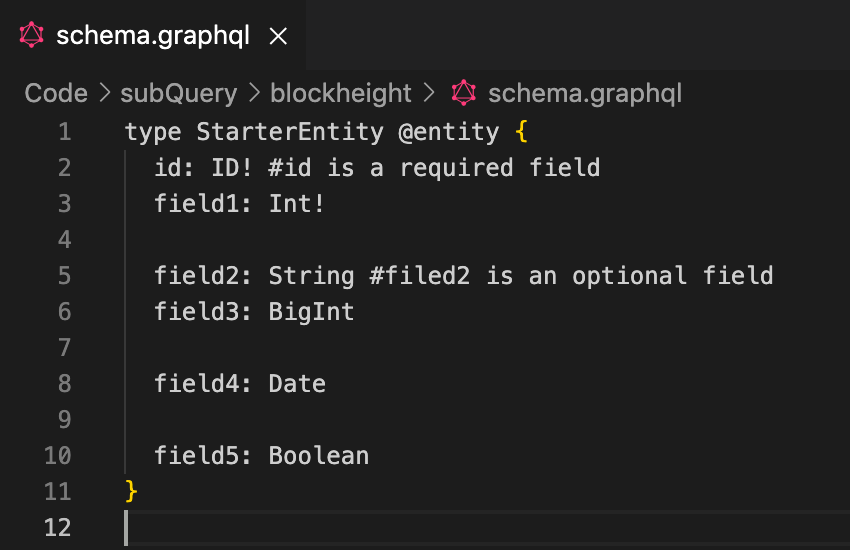
Here, we’ll strip everything down to its bare minimum.
TL;DR
- Delete all the field variables leaving field 1, but rename field1 to blockHeight
- Remove the handleEvent and handleCall from the mappingHandlers.ts to leave only handleBlock.
- Update the project.yaml file to remove the unnecessary mapping handlers (linked to #2).
Pre-requisite
Start by running the standard init command:
subql init --starter myproject
If you are not sure what the above means, check out this.
Step 1: Modify the variables
Change the schema.graph from:
type StarterEntity @entity {
id: ID! #id is a required field
field1: Int!
field2: String #filed2 is an optional field
field3: BigInt
field4: Date
field5: Boolean
}
to
type StarterEntity @entity {
id: ID! #id is a required field
blockHeight: Int!
}
We are a) giving the variable a meaningful name and b) simplifying by removing what is not necessary.
Step 2: Just keep handleBlock
In the mappingHandler.ts file of the default starter project, delete the handleEvent and the handleCall function. We’ll look at these later on. This is what the file should look like.
import {SubstrateExtrinsic,SubstrateEvent,SubstrateBlock} from "@subql/types";
import {StarterEntity} from "../types";
import {Balance} from "@polkadot/types/interfaces";
export async function handleBlock(block: SubstrateBlock): Promise<void> {
//Create a new starterEntity with ID using block hash
let record = new StarterEntity(block.block.header.hash.toString());
//Record block number
record.blockHeight = block.block.header.number.toNumber();
await record.save();
}
Step 3: Update the project.yaml file
The last step is to update the project.yaml file to remove references to the removed functions. It should look like:
specVersion: 0.0.1
description: ""
repository: ""
schema: ./schema.graphql
network:
endpoint: wss://polkadot.api.onfinality.io/public-ws
dictionary: https://api.subquery.network/sq/subquery/dictionary-polkadot
dataSources:
- name: main
kind: substrate/Runtime
startBlock: 1
mapping:
handlers:
- handler: handleBlock
kind: substrate/BlockHandler
Step 4: Build and run the project
Generate and build the code (yarn codegen & yarn build) and then run the project, either in Docker, or have it hosted by SubQuery and then test it with a query. Below is a query you can use for convenience.
{
query{
starterEntities(last:10){
nodes{
blockH
}
}
}
}
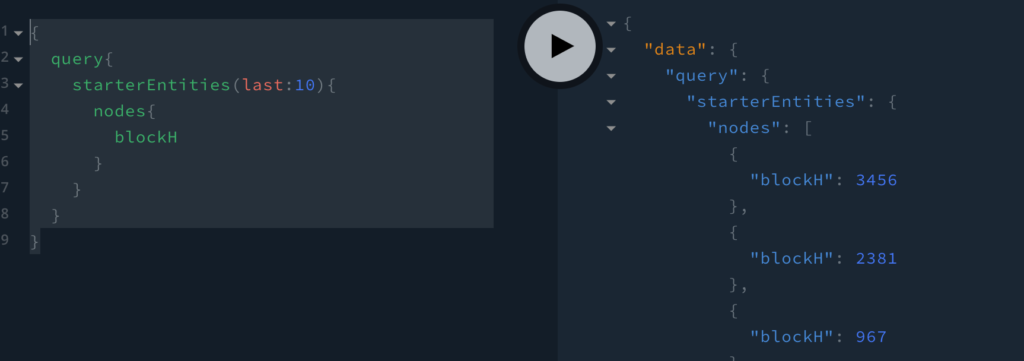